This is part one of a multipart series “Learn Python For SEO“:
Contents: “Learn Python For SEO” is an overview of the course.
Part 1: “Thinking in Algorithms and Writing Pseudocode” ( this article ), Introduces the fundamental programming concepts you will need to get the most out of practical exercises later.
Part 2, “Python – A practical introduction” Gives a hands-on guide to Python using an online Python Interpreter – no complex software installation required
Part 3: “Python – Install Python and Download Your First Website” moves to using a local IDE leveraging Python to download webpages en masse.
Further parts pending…
What is programming?
Let’s start by taking a pragmatic look at how humans use computers to make computer programs.
Computer Programming is the art of preparing a set of instructions which are used by some software running on a computer to produce a set of instructions a computer can understand and guide it to complete a given task.
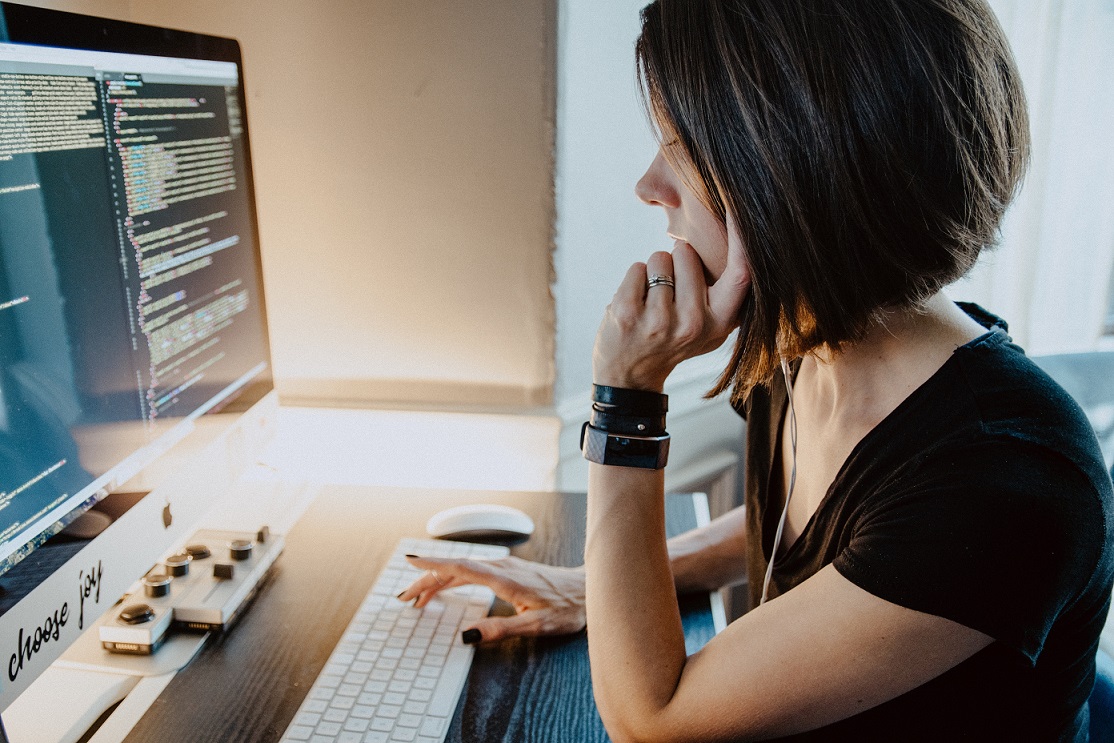
The instructions prepared by the programmer are often expressed in a language that both human and computer can both barely understand. The human needs to learn how to express HOW to complete a set of tasks to the computer, and the computer needs a program to convert the instructions given to it by a human into a set of activities the computer can understand. This is because computers and human beings operate in such a different way, that an intermediary step is required. This intermediary step is fulfilled by programming languages.
There are a variety of Programming Languages out there, and for many
programming languages, there are a variety of “editors”. Editors – and
“Integrated Development Environments” are conceptually similar to
specialised word processors that understand how a language is expressed.
This course will focus on a popular computer programming language –
Python – and take you from first principles into being able to create
your own programs.
Python ( in common with many programming languages ) has a core set
of commands and a structure to follow. We will begin by looking at the
general theory of how languages are put together, and follow on next
time with how to get started in Python.
How do I express problems in a way a computer understands?
It was suggested above that a computer program is “barely understood by both human and computer”. This is because a computer program needs to be expressed in a language understandable by both humans and computers – a “half way house”. As with many forms of interaction – both parties have to compromise a little to make space for the other’s needs.
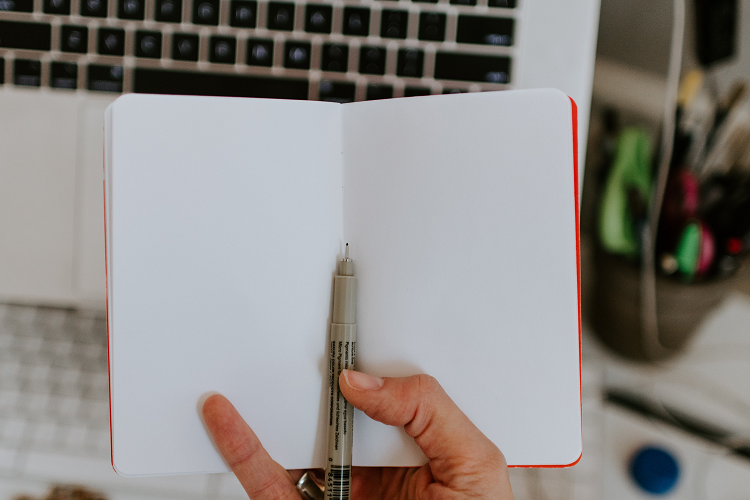
A computer is a very powerful machine, but it requires a great deal of guidance to achieve any given task. A certain form of thinking is required about how we communicate that guidance before we try to program the code. We want to think about algorithm(s) we can use to achieve a task. An algorithm is a structured way of expressing a problem that is understandable by humans, but typically not understandable by machines.
Typically, algorithms are expressed in a form called “pseudocode” – a set of clearly specified steps that a programmer could understand unambiguously, and enter into a computer.
Sometimes, programmers use algorithms and pseudo code when designing programs. Sometimes programmers use these techniques to explain an existing concept to another programmer.
This presents the learner a problem – in order to express an algorithm in pseudo code, we need to understand a bit about programming. In order to understand programming, we need to understand how to express our thoughts in an algorithmic way. To some extent this is a cyclic problem – we get better at writing pseudocode by implementing programs based on pseudocode!
A common way of introducing algorithms to people new to programming is to encourage them to try to engage an engineering way of thinking by looking at a familiar, everyday task, and breaking this down into steps.
There are a number of example algorithms of every day algorithms on the web, of which these are some examples:
- Author Aristides S Bouras presents an Algorithm for making a cup of tea.
- Nathan Seilikoff explains Algorithms in the context of making a sandwich.
Algorithms can be thought of as a set of very basic steps that must be undertaken to achieve a task. Expressing an algorithm in written form often requires the use of pseudo code – an approach to stating the steps needed to achieve the task in a clear and consistent way. We could express an Algorithm for trying to arrange a first time informal meeting with someone as follows :
Given a mutual acquaintance, Discover person to greet’s name Discover a hobby or past time connecting you to the person to greet Think of a contemporary topic around the past time Make Eye Contact Smile Say “Hello, it’s”…, and then add the persons name. continue …“ isn’t it?” Say “I am a friend of ” and then add the name of the mutual acquaintance Say “My name is ” ( add your name here ) Say “I understand from …” ( add friends name here ) Say “… that you are into “ ( add past time ) Say “I don’t suppose you have five minutes ” ( add persons name ) “I’d love your insight into ” ( add contemporary topic )
This may appear a little forced and artificial – that’s ok – it’s
just an example of trying to express some steps to complete an everyday
event. It may be worth thinking of other everyday tasks or chores and
seeing if you can express them as a series of steps which are presented
in a consistent manner.
We can see that the example algorithm above and some of those linked to above have the following common properties:
• We express the problem linearly – the algorithm is a step of steps that proceed from one to the next unless otherwise indicated.
• Each step is simple, and steps requiring similar actions are expressed in a consistent way.
With experience, different people tend to write similar looking pseudocode. That skill comes with time – it’s not necessary to speak algorithms like a developer that’s been coding for twenty years in order to learn how to program. The main thing for now is to try to think of a problem in a way that expresses the steps required for the individual problem in a consistent way.
If you want to read up more on algorithms, there is an excellent guide at BBC bitesize
Beyond Simple ( Linear ) Algorithms – Repetition and Decisions/Choices
The greeting algorithm presented above is regimented, but straight forward. It expresses a problem as a series of steps – one to be followed after the other. However, this approach is only an option for the most simple of problems.
Computers tend to be good at doing achievable things over and over again, or, given some input, doing one of a small number of things to achieve output.
In computing terms, repetition is typically achieved by using Loops – usually in conjunction with some manner of condition that will result in the end of the loop.
A simple way of expressing a Decision or Choice in a computer program or algorithm is a test to see “IF” a certain condition has been reached.
This allows us to express problems in a more computer friendly way. Let’s say that we adapted the “greeting” logic above to a more sensible problem for a computer – such as to send an email asking for a link, or – to make best use of a computer, to send lots of emails asking for a link. Ignoring the bluntness of the approach for a moment, we can then use the concept of a loop to re-write the algorithm.
For this bit of pseudocode, we are trying to be a bit more consistent in how we express the individual steps, and have also sneaked in a new concept – a comment. A comment is a form of documentation that is embedded in the program code. A comment is not intended to form part of the process executed by the computer, but is instead intended to be helpful to the programmer or reader who is trying to understand what the code does. In the pseudocode that follows, lines that are comments will begin “#”.
# Prepare excel spreadsheet of recipient data containing rows of:
# Recipient “email”,
# The recipients “name”,
# The “title” of a recent post they have written on something relevant
Loop Through Each row Of The spreadsheet:
Prepare Email To email address from row
Add Line “Dear” (add “name” from row here )
Add Line “My name is Ann Eggsample,”
Add Line “I loved the post you wrote on ” ( add “title” from row here )
Add Line “It really resonated with me, I was going to comment on your post,”
Add Line “But thought of so much that I ended up writing my own post”
Add Line “I would love it if you could read it and let me know what you think,”
Add Line “or link to it if you think it adds to the valuable and relevant debate”
Add Line “your article started.”
Add Line “”
Add Line “Kind Regards,”
Add Line “ Ann.”
Send Email
End Of Loop.
We can make the above algorithm even more useful by modifying the algorithm above, and adding a choice to tailor the content depending on how old it is:
# Prepare excel spreadsheet of recipient data containing rows of:
# Recipient “email”,
# The recipients “name”,
# The “title” of a recent post they have written on something relevant
# The date the post was written
Loop Through Each row Of The spreadsheet:
Prepare Email To email address from row
Add Line “Dear” (add “name” from row here )
Add Line “My name is Ann Eggsample,”
Add Line “I loved the post you wrote on ” ( add “title” from row here )
If ( “Date of Post” from row is more than two years ago ) Do The Following:
Add Line “It really resonated with me, I was going to comment on your post,”
Add Line “But thought of so much that I ended up writing my own post”
Add Line “It was only then I noticed that your post was over two years old.”
Add Line “”
Add Line “I had a look on your site, but could not find anything more up to date,”
Add Line “if you are working on an update to the post, could you let me know”
Add Line “when it is released so I can link to it.”
Add Line “”
Add Line “I think it would help your users if you linked from your post”
Add Line “to my post in the meantime, but I will leave that to you.”
Add Line “”
Add Line “Look forward to hearing from you,”
Add Line “ Ann.”
Otherwise If ( “Date of Post” from row is more recent ) Do The Following:
Add Line “It really resonated with me, I was going to comment on your post,”
Add Line “But thought of so much that I ended up writing my own post”
Add Line “I would love it if you could read it and let me know what you think,”
Add Line “or link to it if you think it adds to the valuable and relevant debate”
Add Line “your article started.”
Add Line “”
Add Line “Kind Regards,”
Add Line “ Ann.”
End Of If Choice
Send Email
End Of Loop
We now have pseudo code for an algorithm to send custom outreach emails to a number of recipients based on the content – we are designing software!
So far, we have looked at:
1) A programming language as a compromise between what the human and computer can understand
2) Expressing a problem as an algorithm consisting of a number of steps
3) Writing an algorithm using pseudocode
4) Loops
5) Decision making based on Conditional “If” choices
There are two more key programming concepts to introduce before we move on to look at how to write and execute Python code:
6) How a programming language expresses remembering things.
7) How we can break up a big bit of code to make it more readable.
Variables
In this section we will look at how we express the need to remember things in a programming language.
Modern Computers are able to store lots and lots of data, programs
and pictures. Computer programmers need ways of manipulating the
information held within a computers store, or memory.
All
information used by a computer program needs to be stored somewhere –
even if the information is stored for a short period of time. This
concept is true of all sorts of information – including big things like
movies, photos, or spreadsheets and little bits of data like the mutual
acquaintances name in the first example.
We will look at interacting with big pieces of information like spreadsheets later in this course, but can start with a look at how a computer program can store and manipulate the name of an individual, by looking at a very simple piece of pseudocode to greet a user of the program.
With this example, we will introduce a subtle nuance with comments – let’s give ourselves the option to add them at the end of step of pseudo-code – so that anything after “#” on a line is for human eyes only, and if there is anything before a “#” on a line, that is intended to be a step the computer performs.
# Pseudocode to greet a user Show Message “What is your name?” # somehow get and store name here Show Message “Hello ” # somehow add name of user here
Many programming languages have the concept of “input” – the process of asking a user for data. However, as we discussed above, the information needs to be stored somewhere. Programming languages try to make this storage of small bits of information easy by using “variables”.
“Variables” act as virtual boxes which contain the small bit of information.
Variables are a very commonly used concept in programming. Because of this, programming languages tend to make variables easy to create, access and modify.
Different programming languages impose different rules on how to create and name variables – for the impatient and curious, there are a number of documents online describing the rules python has for naming variables .
For now, in pseudocode, we could suggest that a variable can be represented by any word that’s all in lower case – an exception being comments, or the phrases above which are intended to be shown or sent to the user and are quoted in speech marks.
As our variable is to store the name of the user – we will call our variable “name”. We will express the ability to get input from the user using “Input”, and will express the transfer of the value received from the user into the variable called name using the equals sign.
We can then rewrite our short “Hello User” pseudocode to be:
# Pseudocode to greet a user Show Message “What is your name?” name = Input Show Message “Hello ” # somehow add name of user here
Our last issue with this bit of pseudocode – how should we express the inclusion of the name of the user from the variable “name” into the greeting?
For now – to keep our pseudocode simple – let’s suggest that we will express the desire to include a variable into a message for a user by surrounding the variable name with curly brackets, thus:
# Pseudocode to greet a user Show Message “What is your name?” name = Input Show Message “Hello {name}”
Our pseudocode is starting to look like a real programming language – a set of steps, with consistent names, and the creation of a variable to hold user input, which is subsequently used to display the user input back to the user.
Functions
The last concept we are going to look at in this session is “How we can break up a big bit of code to make it more readable?”
So far, we have expressed pseudocode as a set of linear steps that could be performed in order to achieve a goal. We have suggested that pseudocode is a way of expressing a problem that a programmer could pick up and use as guidance on how to solve a problem.
Simple sets of linear steps – like the pseudocode we have looked at so far, are good for some problems. However, this approach of expressing code as long list of linear steps suffers from two problems:
1) Some problems require the same sets of small steps to be repeated in a way that is more complex than a simple loop.
2) Sometimes expressing all the steps linearly makes the program more difficult to understand.
A solution to both of these problems are “Functions”. Functions are a set of steps that are not processed straight away, but instead form a library of code that can be called when needed.
A Function consists of three features – a name, a set of steps, and something we will explain later called parameters.
Let’s look back at our email algorithm above, and remove some code to make it more readable:
# Prepare excel spreadsheet of recipient data containing rows of: # Recipient “email”, # Their “name”, # The “title” of a recent post they have written on something relevant # The date the post was written Loop Through Each row Of The spreadsheet: If ( “Date of Post” from row is more than two years ago ) Do: # send the old post email Otherwise If ( “Date of Post” from row is more recent ) Do: # send the new post email End Of If Choice End Of Loop.
We have hidden the implementation details of sending the old post and new post emails, hopefully making the logic clearer as a result. We can use functions to implement sending the old post email and sending the new post email.
How do we create such a Function? Because Functions are a common feature in programming languages, they are easy to create – almost as easy as variables. We can mirror this ease of declaration in our pseudocode by choosing to declare functions in the format:
Function function_name: # Step 1 for this function # Step 2 for this function # Step 3 for this function # Step 4 for this function # Any more steps for this function … End Function
We have continued the format used above of marking the end of a Function. We have used “End Of Loop” and “End Of If Choice” above, but as we are making the rules of our pseudocode, but we need to be consistent, we can trim down some of the verbosity. Therefore, instead of using “End Of Function”, we can just use “End Function”.
In order to express how we access the steps in the function and perform them, we can adopt the form:
Call Function function_name:
Or just simplify to:
Call function_name:
An important thing to note – this is our pseudocode – we could choose different words for defining and executing Functions as long as we are consistent with how we use our language. We could express the concept of calling a function by instead choosing “Execute function_name”, “Bosh function_name” or even something as subtle as adding open and close brackets after the function name like “function_name()”. The important factor is that we are consistent in the way we express calling a Function within our pseudocode.
When we looked at variables, we suggested that we needed a rule so we knew what a variable looks like and what a step was. We suggested that a variable would be all lower case, and that instructions in the steps would start with an upper case letter. For now, we will expand the suggestion that variables are lower case words to include lower case letters and the underscore character – so we can have variable_names that are more than one word long. We will and can borrow this variable naming rule and use it for naming functions as well.
We can now add the text of the email back to our format, like this:
# Prepare excel spreadsheet of recipient data containing rows of:
# Recipient “email”,
# Their “name”,
# The “title” of a recent post they have written on something relevant
# The date the post was written
Loop Through Each row Of spreadsheet:
If ( “Date of Post” from row is more than two years ago ) Do: <br>
Call old_post_email
Otherwise If ( “Date of Post” from row is more than two years ago ) Do:
Call new_post_email
End If
End Loop
Function old_post_email:
# Prepare Email To ( email address from row )
Add Line “Dear” (add “name” from row here )
Add Line “My name is Ann Eggsample,”
Add Line “It really resonated with me, I was going to comment on your post,”
Add Line “But thought of so much that I ended up writing my own post”
Add Line “It was only then I noticed that your post was over two years old.”
Add Line “”
Add Line “I had a look on your site, but could not find anything more up to date,”
Add Line “if you are working on an update to the post, could you let me know”
Add Line “when it is released so I can link to it.”
Add Line “”
Add Line “I think it would help your users if you linked from your post”
Add Line “to my post in the meantime, but I will leave that to you.”
Add Line “”
Add Line “Look forward to hearing from you,”
Add Line “ Ann.”
Send Email
End Function
Function new_post_email:
# Prepare Email To ( email address from row )
Add Line “Dear” (add “name” from row here )
Add Line “My name is Ann Eggsample,”
Add Line “I loved the post you wrote on ” ( add “title” from row here )
Add Line “It really resonated with me, I was going to comment on your post,”
Add Line “But thought of so much that I ended up writing my own post”
Add Line “I would love it if you could read it and let me know what you think,”
Add Line “or link to it if you think it adds to the valuable and relevant debate”
Add Line “your article started.”
Add Line “”
Add Line “Kind Regards,”
Add Line “ Ann.”
Send Email
End Function
We now have a reasonably clear statement of intent that explains the algorithm in pseudocode that sets out the steps that need to be taken to send appropriate emails out for this outreach activity, in a way that uses Functions to separate the logic of choosing which email to send from the sending of each type of email. There are some steps that are expressed in English rather than pseudocode ( such as “( add “title” from row here )”. This use of English isn’t a problem for pseudocode – if anything, it is a nice “tuit” to help us think about how we can express this better, or to research how these areas are expressed in computer language, so we can enrich the vocabulary of our pseudocode to make it capable of expressing more complex concepts..
We have one remaining loose end. We have introduced a way of expressing the steps a Function has, and a way of naming Functions, but we promised to discuss a third aspect of “parameters” earlier.
A subtly with the pseudocode above is that the new_post_email and old_post_email Functions both need to know which row is being processed in order to work. In the pseudocode above, this is assumed and hence implicit. When writing computer programs, it is considered good practice to explicitly inform a Function of any data it needs to function.
In practice, this is often achieved using a special form of variable called a Parameter. A Parameter exists to pass data into a Function, so that a set of steps can make use of the information passed into the Function when it is called.
Passing data into Functions using Parameters is very powerful as it this means the same input steps can be applied to different bits of data.
So how do we use Parameters? Parameters can be listed as part of a Functions declaration, and then subsequently used in the steps contained within a Function. Let’s explore this concept in practice by adapting the greeting pseudocode we used to introduce variables to make it greet five friends:
# Pseudocode to greet five friends greet(“bob”) greet(“sue”) greet(“rita”) greet(“jeff”) greet(“andy”) Function greet ( name ): Show Message “Hello {name}” End Function
Functions and Parameters are essential parts of programming, but do add a level of complexity. If you aren’t confident you fully understand Parameters yet, don’t worry. We will come back to Parameters and Functions in future sessions.
Wrapping things up.
In this post, we’ve looked at some general concepts of computer programming. We have looked at the role of a programming language, suggested a process to developing software, and examined some building blocks of programs
What is Computer Programming?
Humans tend to tell Computers how to tackle problems by using
computer programs. A program is a set of steps a computer needs to
perform that is written in a language understandable by both human and
machine.
We need to think about a problem in a certain way to be able to express the steps needed for a computer to solve the problem.
If we can think of a series of steps that are needed to achieve a task,
in a structured way, then we call that structured set of steps an
Algorithm. We can express Algorithms in “pseudo code” – a set of
consistent steps that looks a bit like a program but is not executable
by a computer.
A simple process for developing software:
1) Find an opportunity or problem you want a computer to solve
2) Think about how to solve the problem algorithmically
3) Express the problem solving Algorithm in pseudo code
4) ( To come ) Convert the pseudo code into a program
Building blocks of computer programs
Concept | Explanation |
---|---|
Algorithm | A set of steps which express how to solve a problem. The steps should be expressed in a way that is consistent and understandable to programmers |
Choices/Decisions | The ability for a program or algorithm to execute a set of steps if a condition is met |
Loop | A way of expressing that certain steps should be repeated until some condition(s) are met or some data is exhausted. |
Function | A set of steps that are not processed immediately, but instead, are remembered, and can be used one or more times by the program or algorithm. A Function has a name and (optionally) some parameters which can be used to pass information into it. |
Variable | A named store where small bits of information can be remembered |
Next time, we will introduce Python, and look at moving from Pseudocode and Algorithms to real programs!
This is part one of a multipart series “Learn Python For SEO“:
Contents: “Learn Python For SEO” is an overview of the course.
Part 1: “Thinking in Algorithms and Writing Pseudocode” ( this section ), Introduces Algorithms and Pseudocode.
Part 2, “Python – A practical introduction” Gives a hands-on guide to Python using an online Python Interpreter – no complex software installation required
Further parts pending…
- Introducing Duplicate Link Detection - August 27, 2021
- Python – A practical introduction - February 25, 2020
- Get a list of pages on your site with links from other sites. - February 7, 2020